Why should you generate code from your schemas?
Generating code from your GraphQL schemas can help improve your development workflow. By doing so, there's less amount of code you'd need to write, which in turn saves you time and is easier to maintain. You can detect errors when there are changes to the schema, making the codebase less error prone.
How can you use your schemas to generate code?
Initial Setup
Start off with creating a basic React app using typescript.

Generating code from your GraphQL schemas can be done using
graphql-code-generator. Using this tools, you're able to configure it in many different ways to fit your needs and workflow.
For this example, I’ll be using it to generate code for
React JS, Typescript, and Apollo.
Add graphql and graphql-code-generator packages:

Update package.json:

Using Github GraphQL API
We’ll need a GraphQL backend server to query against. I’ll be using Github’s GraphQL API. Download Github's
schema.docs.graphql file and place it at the root of the project to be used with code generation.
Add codegen.yml to the root of the project.

- schema: can be set to point to wherever you have your GraphQL schema located. It can be set to point to a backend GraphQL server so that whenever you generate code they’re in sync with the server. Here it's set to read locally from our schema file.
- generates: is set to output code to ./src/generated/graphql.tsx.
Generate code from the Github schema by running:

Inspect ./src/generated/graphql.tsx, you’ll see all the types that were created from the schema. In a matter of a few seconds, all of it was created for you saving you time from having to manually create them. You don't have to maintain any typings you'd have to write yourself. Any changes to the schema can be regenerated and reflected back in the code. It also gives you the benefit of detecting errors early on if there was a change to the schema and a mismatch in typings when generating code from the schema.
Adding Apollo Client
Now we’ll need a GraphQL client to make queries to the server. We'll use Apollo client for that and need to configure it to generate code to use Apollo.
All apollo client and typescript-react-apollo:
Update codegen.yml:
- documents: is set to look for any GraphLQ files within the directory to be used for code generation.
- withHooks: we’ll also set the configuration to only generate hooks that we can use.
Additional configurations can be found
here.
Query Github API
Create a graphql file src/queries/CurrentUser.graphql:
Then we’ll run yarn generate
to generate the hook for the query. Inspect src/generated/graphql.tsx and you should see that it generated this portion of code you can use.
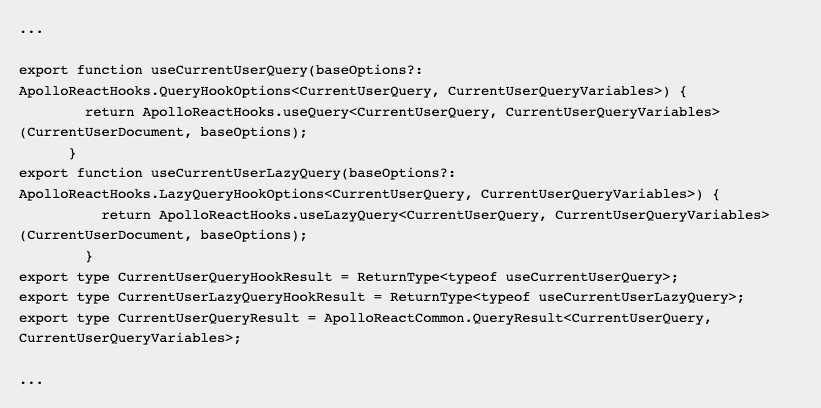
Next we’ll need to setup the apollo client.
Add src/apolloClient.ts
You’ll need a personal access token from GitHub that can be obtained by follow these
instructions. Once you have your token, replace
<GITHUBACCESSTOKEN> with it.
Next we’ll add ApolloProvider in src/index.tsx.
That’s it for setting up Apollo. Next we'll update src/App.tsx to use the hook to query our data.
Import useCurrentUserQuery from ./generated/graphql and display the data.
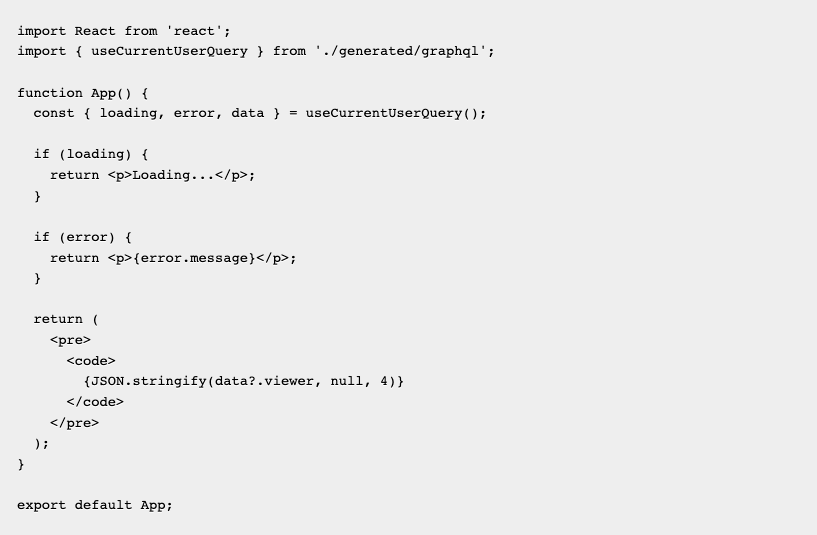
Viewed in the browser results in:
Next we’ll update src/queries/CurrentUser.graphql with some additional data.
Then run yarn generate
and refresh the page. The code will reflect the new schema changes and the page should display the new information.
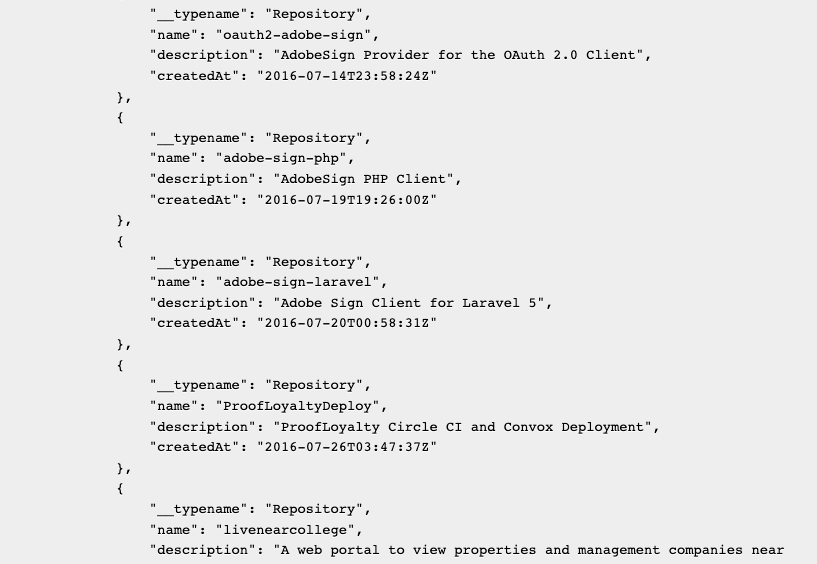
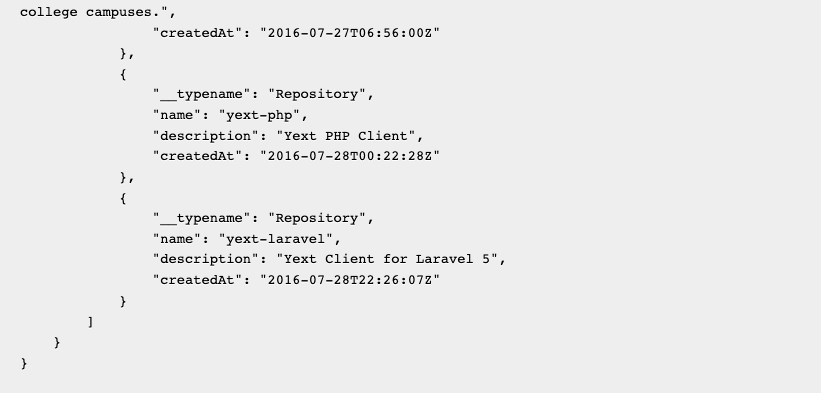
Conclusion
Consider generating code from your GraphQL schemas in your next project. It can improve your development workflow by having less code you’d need to write which is less code you’d need to maintain and in turn would be less error prone. You can checkout the full source code
here.