Gatsby Starter (with Typescript)

gatsby-plugin-typescript
is automatically included in Gatsby. Only explicitly use this plugin if you need to configure its options.Continuously Build and Deploy Site on Netlify
Trigger builds from changes in code base
gatsby-cli
(gatsby new gatsby-site) or one of the many flavored starters, create a Git repo and push all local changes to remote. Then follow the instructions to link your repo in Netlify Console after you have authorized Netlify through Github account (or other platform of your choosing). One thing worth noting is that Netlify allows you to deploy multiple branches. This comes useful when you have both production
and staging
environment.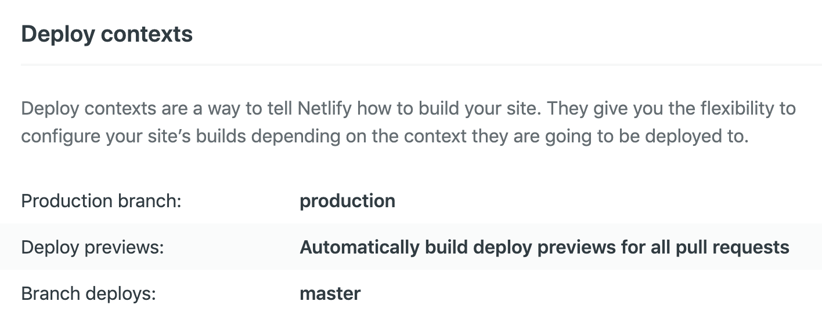
master
, set up as staging branch) and deployed to branchname--yoursitename.netlify.app
.Trigger builds from changes in data source
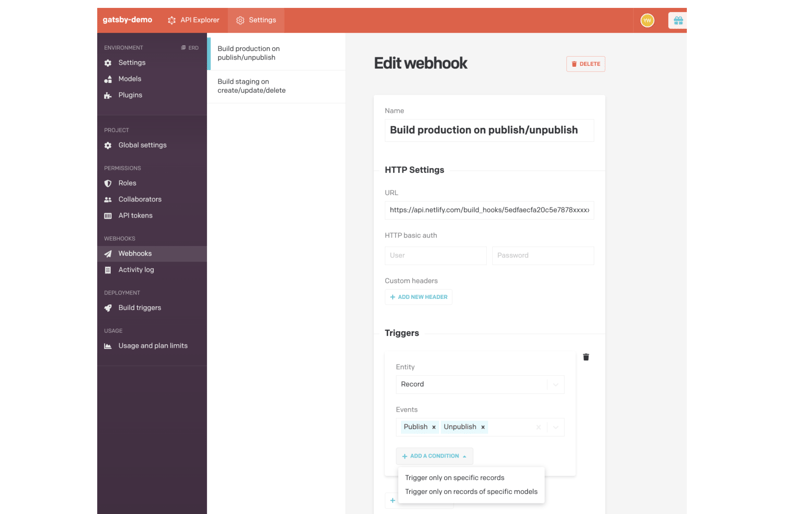
Runtime vs. Build Time
gatsby develop
spins up browser for you, meaning you can make reference to the window object without triggering any error. Running gatsby build
will generate optimized assets, however, the browser does not exist in build time so using "browser globals" like window or ducument object without first checking its existence will trigger a build error. To fix this, either check if the browser globals are defined; or, if the code is in render function of a React JS component, move that code to a componentDidMount() lifecycle method / useEffect hook.Sourcing Data with plugins, Querying Data with GraphQL
BYOD (Bring-Your-Own-Data)
gatsby-source-filesystem
plugin), Databases (gatsby-source-mongodb
plugin), Headless CMS (gatsby-source-datocms
, which we will be using in the example), and so on.gatsby-config.js
file: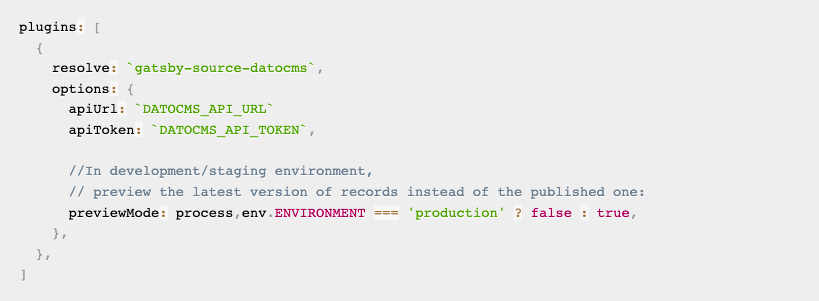
The really novel idea: GraphQL at build time
gatsby-node.js
file using pageQuery
component, StaticQuery
component, or useStaticQuery
hook. However, you cannot mix page queries and static queries in the same file, or have multiple page queries/static queries.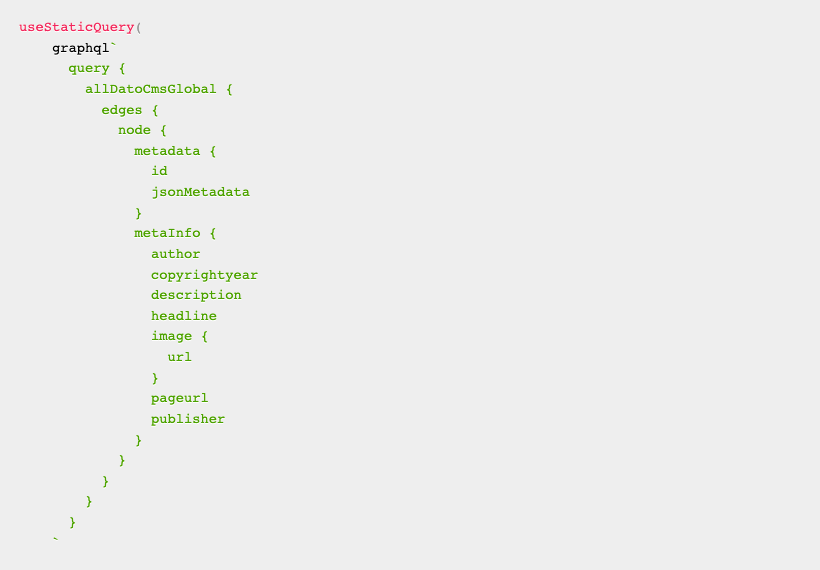
Gatsby Is Not Just for Static Site
React Hydration makes "app-like" features possible
ReactDOM.hydrate()
is called internally by Gatsby from ReactDOM
. The method is the same as render()
but is used to hydrate HTML contents initially rendered by ReactDOMServer
. This allows for React to pick up whatever is left after static site generation, then you can do your dynamic data fetching at runtime.-
Static pages
- Automatically create pages throught
src/pages
- Programmatically with
createPage
API
- Automatically create pages throught
- Hybrid app pages Statically created pages can make calls to external services and third party APIs for dynamic data-fetching. These pages are referred as hybrid app pages.
- Client only routes Using a client-side router such as
react-router
or@reach-router
(@reach-router is what Gatsby uses behind the scene, so no need to install it), you can create page by exporting a component inside pages directory, or import a router and set up routes yourself.
Expand Site To Be Even More Dynamic
Add Netlify Functions to Gatsby

netlify.toml
file at the root of your repositorygatsby build
must be run before netlify-lambda build src/functions
or else the Netlify function builds will fail.
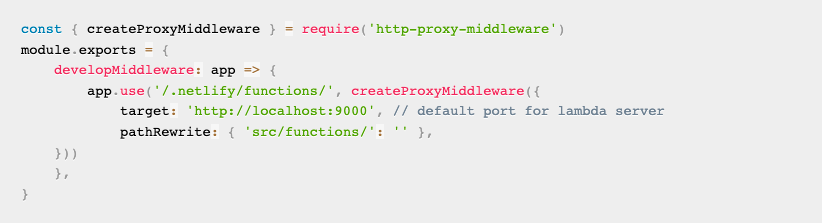
/src/functions
(path specified in netlify.toml
file), then access from your Gatsby app like this: fetch('/.netlify/functions/[YOUR_FILE_NAME]').then(() => ())
netlify.toml
fileAdd Authentication in Gatsby
- Gatsby statically renders all unprotected routes as usual
- Authenticated routes are whitelisted as client-only, wrapped in an authentication provider
- Logged out users are redirected to the login page when they attempt to visit a protected route
- Logged in users will see their private content
Use Netlify Identiy

/dashboard
will be handled by client-side app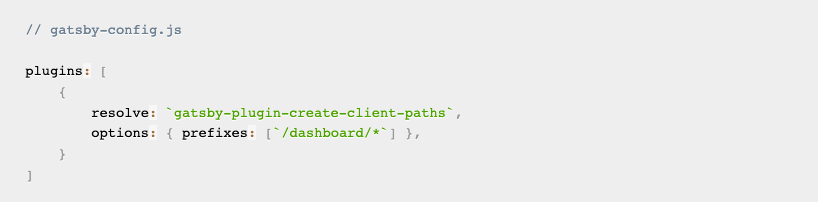
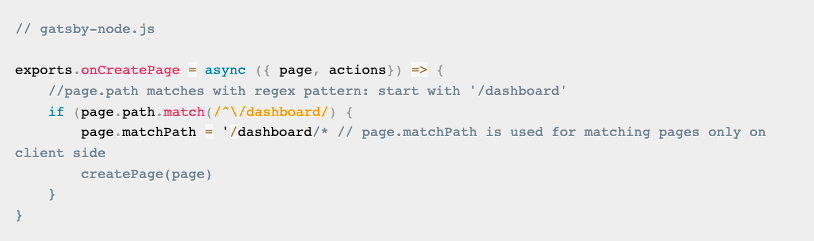
5. Wrap with the Provider
Unlike create-react-app
application, Gatsby does not come with App.tsx
file but we can wrap our CustomContext.Provider
and ThemeProvider
using wrapRootElement(). Then we export wrapRootElement() in both gatsby-browser.js
and gatsby-ssr.js
. Without wrapping the root element in both files there will be mismatch betwee client and server side output.
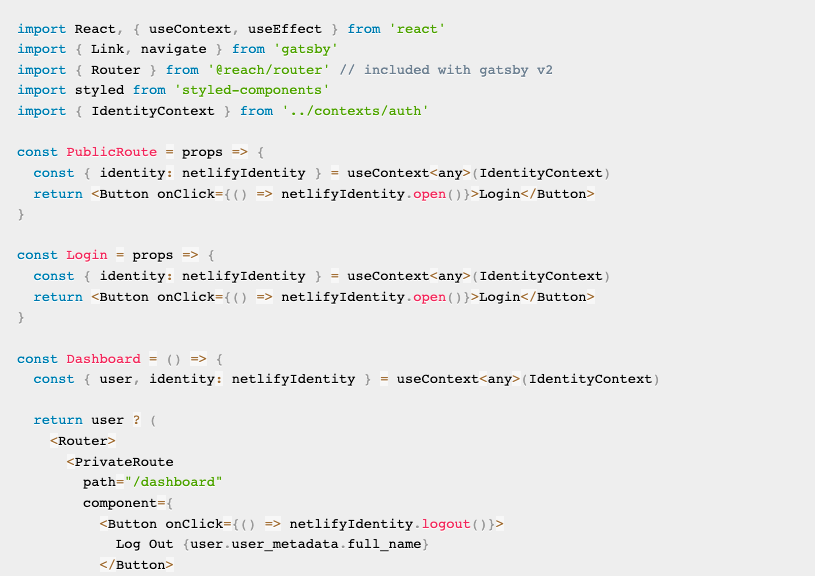
Use Authenticated Netlify Functions with Netlify Identity
context
object.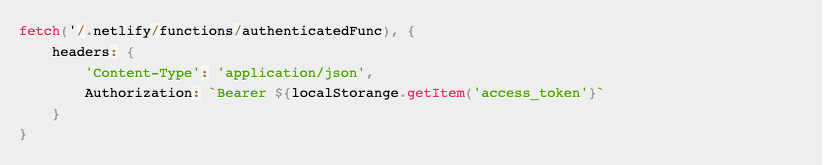
user
object is present if function request has Authorization header like below:Authorization: Bearer <access_token>
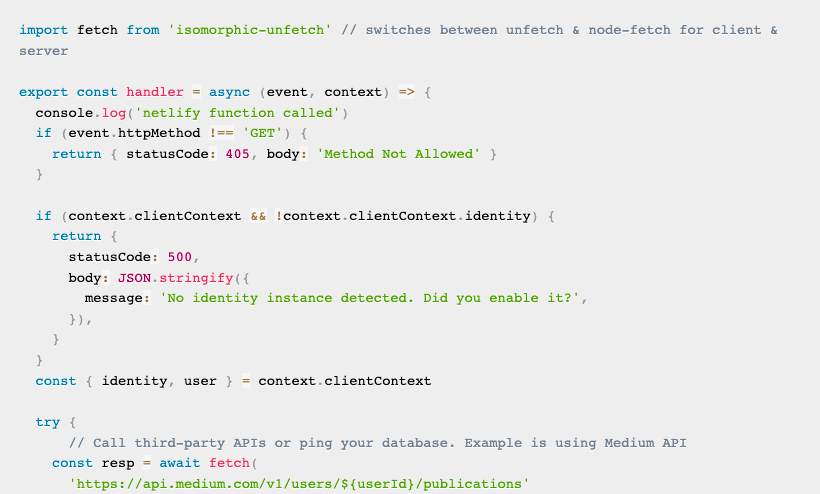
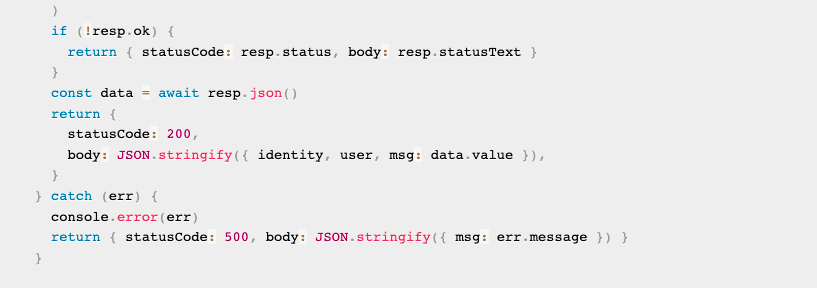